Get WooCommerce product variation price and sale price for your pricing table
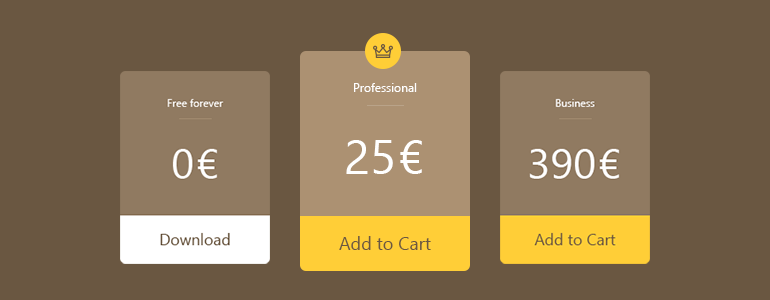
So as usual I was peacefully working on a project of my own using WooCommerce as a base of my e-commerce solution. I had previous experience building standard shops consisting of multiple products with variable prices for each variation. But now I was creating a website with a single downloadable product that had multiple variations and each variation a custom price.
Now on the main product page I had to create a pricing table for this variable product to show off different pricing plans. And this is where I came across a problem of how to separately retrieve or get WooCommerce product variation price and sale price. I know that it sounds like an easy task and I assumed that WooCommerce definitely would have prepared such a function but I was mistaken.
In order to display custom product variation price I created a function with 2 attributes:
- $product_id – The ID of the variable product
- $variation_id – The ID of the variation that you need to get the price
So here is the function. You should add this in your functions.php file:
// Get Woocommerce variation price based on product ID function get_variation_price_by_id($product_id, $variation_id){ $currency_symbol = get_woocommerce_currency_symbol(); $product = new WC_Product_Variable($product_id); $variations = $product->get_available_variations(); $var_data = []; foreach ($variations as $variation) { if($variation['variation_id'] == $variation_id){ $display_regular_price = $variation['display_regular_price'].'<span class="currency">'. $currency_symbol .'</span>'; $display_price = $variation['display_price'].'<span class="currency">'. $currency_symbol .'</span>'; } } //Check if Regular price is equal with Sale price (Display price) if ($display_regular_price == $display_price){ $display_price = false; } $priceArray = array( 'display_regular_price' => $display_regular_price, 'display_price' => $display_price ); $priceObject = (object)$priceArray; return $priceObject; }
And after you have added the function you can easily use it to display WooCommerce product variation regular price an variation sale price on your website. Add this code where you want the prices to be displayed. Change 9 to your product ID and 15 to your product variation ID.
<?php $variation_price = get_variation_price_by_id(9, 15); echo $variation_price -> display_regular_price; echo $variation_price -> display_price; ?>
If you are wondering where to get ID values of variations then you can take a look in the screenshot below. Product Variation ID values are right next to variation labels.
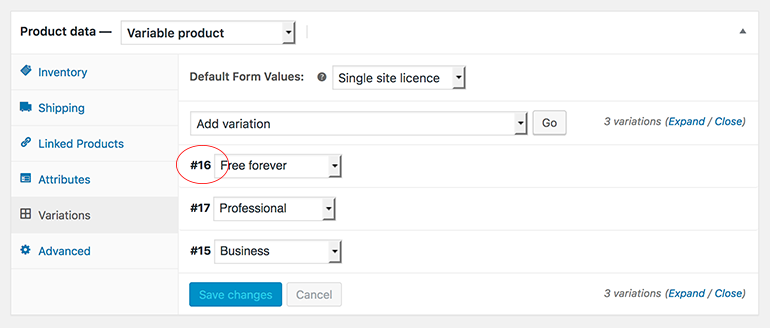
Now you will be able to create custom pricing tables using Wordpres and WooCommerce and will not have to worry if you update product variation prices – they will be also updated and displayed in the pricing table. Please let me know your thoughts below and if you would like to get some extra information about the product variations.
You’re a life saver man! Tried so much before I stumbled upon this. This is perfect :)
Great to hear that it helped :) Cheers!
Thank you so much, it worked perfectly :D
Glad it helped you, Kiara :)
Great tutorial, only one question.
Is it possible to make this line:
variation_price = get_variation_price_by_id(9, 15);
dynamic?
So Product ID and variation ID are filled out automaticly like:
variation_price = get_variation_price_by_id($product->ID, $variation->ID);
Hey Joep,
I think it is possible but I only required a very specific product to be customized like this.
If you would like to do this using dynamic values, I am guessing you would have to define $product as global and then get an ID from it:
global $product;
$product_id = $product->get_id();
And this is how you can get product variations:
$available_variations = $product->get_available_variations();
This is just a direction to go next, but hopefully it helps you.
Hello,
This post is good, very nice work
Thank you
Hi this is cool, im looking for help trying to show some prices for EMI plans on the pp.
/*** start – show instalment price on product page***/
add_action( ‘woocommerce_single_product_summary’, ‘show_inst_price’, 30 );
function show_inst_price() {
global $product;
$product_id = $product->get_id();
$product = wc_get_product( $product_id );
$price=$product->get_price();
if( $price > 20000 ){
$sey36 = 2.849; // bank convenience ‘%’
$multiplysey36 = $price * $sey36;
$sey36price = $multiplysey36/$percentage;
echo ‘0% interest instalments starting from Rs. ‘ . number_format($sey36price) . ‘/month‘;
so this echos a calculation of $price, which is the lowest price of variation, i need $price to be dynamic, so when customer selects the variable price, his price will be updated on the div.
this
Hi Abdur,
Thanks for your question. A bit hard to understand what exactly is going on in your code and therefore to help you out.
Will result in an error.
I have searched everywhere for a solution to getting the variation price output. This code doesn’t work for the newest version of WP + Woo. Could you please update the code? I am desperate to find a solution to my problem :) Cheers Martin
Hey Martin, thanks for getting in touch.
I just tested the code and found there was a small issue in the code, now it’s been fixed and works with both with the latest WordPress and WooCommerce versions.
Hey Nauris, thanks for this very clear article.
Quick question: which link do you put for the add-to-cart buttons ? How does the system know that you are clicking on the “add-to-cart” for 25 euros ?
Thanks,
Caroline
Hey Caroline,
A very good question :)
I guess the easiest way would be to simply manually ouptut an Add to Cart button under each link like so:
<a href="www.yourstoreurl.com/checkout/?add-to-cart=9&variation_id=16">Add to Cart</a>
You see there in the link number 9 represents product ID (make sure to change it according to your Product ID) and then simply make sure to add the correct variation ID value.
Hope this helps.
Hi Nauris!
Thanks for sharing this solution.
I have copied de php code on my functions.php page and try to put the code in a code section on Divi, but I got this:
display_regular_price;
echo $variation_price -> display_price;
?>
What I have done wrong? Thank you.
Hi Marlon, not really sure how Divi code section works, but I would suggest checking if you have everything in place with opening and closing php tags . Maybe they are not required where you are using them.
Hi Nauris,
This is perfect and it worked for me !
But there are a few things hope you suggest to me. I want to format currency.
Ex: The price displayed is: 1234567$ and i want it display to: 1.234.567 $
many thanks !
Hi Kenbo,
This is how you can change the number formatting according to your needs:
I am in a very difficult situation. Please help me. The price of Variated products does not appear in the store. When I go to the product detail page and select the variation; The price does not change depending on the option. An error message appears when I press the add to cart button.
Link: https://www.deneme.website/magaza/
Product with variations: Angora Ametis 2404A
A lot of sites have shared code but it doesn’t work. The price does not change according to the variation. the variation does not trigger the price.
Hi Metin,
Please take a look at this guide on setting up WooCommerce variation prices according to selected variation (e.g., color, size) https://iconicwp.com/blog/display-the-variation-price-in-woocommerce/
Nice, but your code do not show zero if price is for example: 24.90, then it shows only 24.9. There is no problem with 24.99 but if zero is last then you will see only 24.9 – do you have any idea how to fix that?
To always output 2 decimals, you can make small adjustments inside the get_variation_price_by_id() function.
Replace this:
$variation['display_regular_price']
with this:number_format( (float) $variation['display_regular_price'], 2, '.', '' );
And replace this:
$variation['display_price']
with:number_format( (float) $variation['display_price'], 2, '.', '' );
awesome, thank you very much for sharing this useful function :)